With this PR, when JS is activated and WASM supported, the article editor will be dynamically replaced with `contenteditable`s elements. This makes the editing interface simpler and less like a regular form. It will also allow us to easily add visual formatting with native browser APIs (and to insert images or videos directly). Here is a little demo: 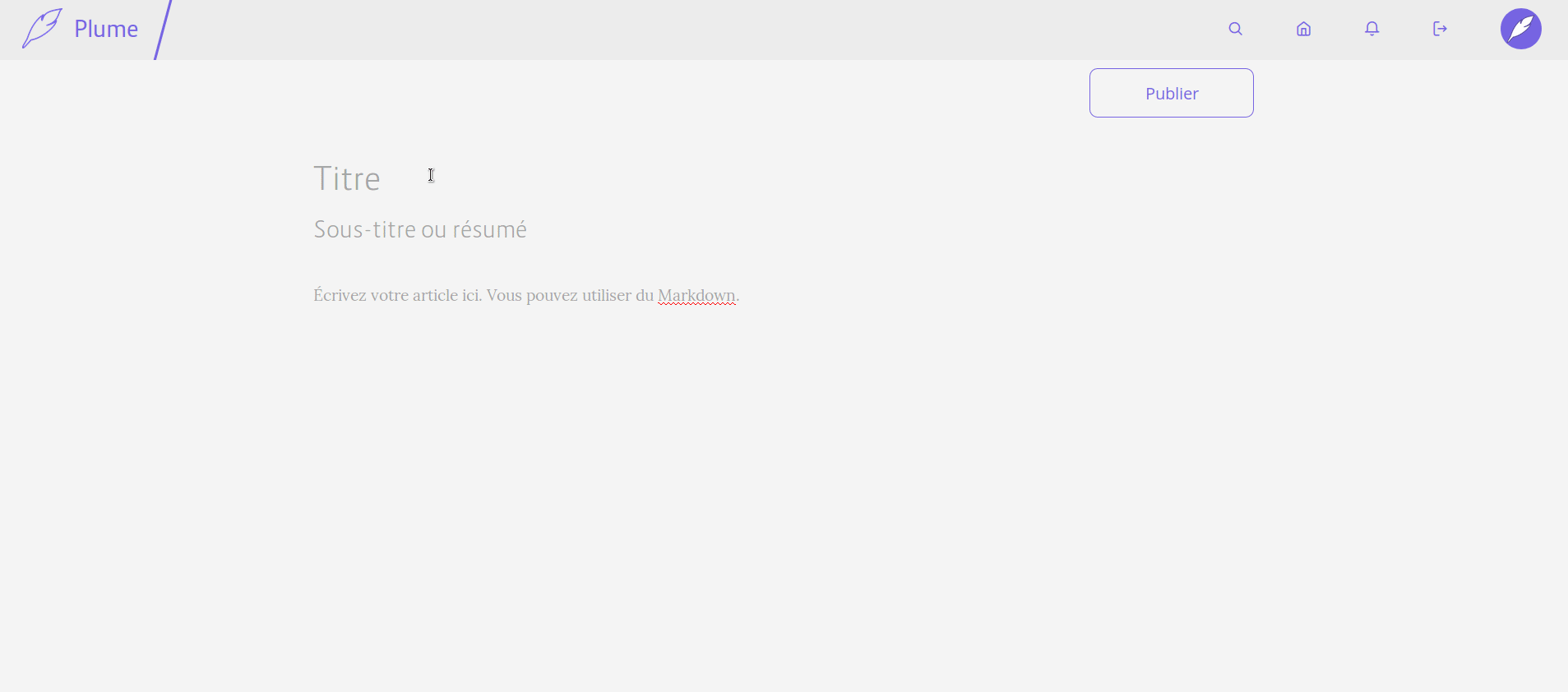 There is still a lot to do, but it is a good first step. Fixes #255
75 lines
2.2 KiB
Rust
75 lines
2.2 KiB
Rust
#![recursion_limit="128"]
|
|
#![feature(decl_macro, proc_macro_hygiene, try_trait)]
|
|
|
|
extern crate gettext;
|
|
#[macro_use]
|
|
extern crate gettext_macros;
|
|
#[macro_use]
|
|
extern crate lazy_static;
|
|
#[macro_use]
|
|
extern crate stdweb;
|
|
|
|
use stdweb::{web::{*, event::*}};
|
|
|
|
init_i18n!("plume-front", en, fr);
|
|
|
|
mod editor;
|
|
|
|
compile_i18n!();
|
|
|
|
lazy_static! {
|
|
static ref CATALOG: gettext::Catalog = {
|
|
let catalogs = include_i18n!();
|
|
let lang = js!{ return navigator.language }.into_string().unwrap();
|
|
let lang = lang.splitn(2, '-').next().unwrap_or("en");
|
|
catalogs.iter().find(|(l, _)| l == &lang).unwrap_or(&catalogs[0]).clone().1
|
|
};
|
|
}
|
|
|
|
fn main() {
|
|
menu();
|
|
search();
|
|
editor::init()
|
|
.map_err(|e| console!(error, format!("Editor error: {:?}", e))).ok();
|
|
}
|
|
|
|
/// Toggle menu on mobile device
|
|
///
|
|
/// It should normally be working fine even without this code
|
|
/// But :focus-within is not yet supported by Webkit/Blink
|
|
fn menu() {
|
|
document().get_element_by_id("menu")
|
|
.map(|button| {
|
|
document().get_element_by_id("content")
|
|
.map(|menu| {
|
|
button.add_event_listener(|_: ClickEvent| {
|
|
document().get_element_by_id("menu").map(|menu| menu.class_list().add("show"));
|
|
});
|
|
menu.add_event_listener(|_: ClickEvent| {
|
|
document().get_element_by_id("menu").map(|menu| menu.class_list().remove("show"));
|
|
});
|
|
})
|
|
});
|
|
}
|
|
|
|
/// Clear the URL of the search page before submitting request
|
|
fn search() {
|
|
document().get_element_by_id("form")
|
|
.map(|form| {
|
|
form.add_event_listener(|_: SubmitEvent| {
|
|
document().query_selector_all("#form input").map(|inputs| {
|
|
for input in inputs {
|
|
js! {
|
|
if (@{&input}.name === "") {
|
|
@{&input}.name = @{&input}.id
|
|
}
|
|
if (@{&input}.name && !@{&input}.value) {
|
|
@{&input}.name = "";
|
|
}
|
|
}
|
|
}
|
|
}).ok();
|
|
});
|
|
});
|
|
}
|